Tuesday, October 1, 2019
Wap to display 1,2,3,5,8, up to 13th term
Wap to check whether the given number is perfect square or not
CLS
INPUT "ENTER ANY NUMBER"; N
S = SQR(N)
PR = PERFECT (S)
IF PR = S THEN
PRINT "PERFECT SQUARE"
ELSE
PRINT "NOT PERFECT SQUARE"
END IF
END
FUNCTION PERFECT (S)
PERFECT = INT (S)
END FUNCTION
Wap to find whether the given number is positive negative or zero
CLS
INPUT"ENTER ANY NO.";N
CALL CHECK(N)
END
SUB CHECK(N)
IF N>0 THEN
PRINT"NUMBER IS POSITIVE"
ELSE IF N<0 THEN
PRINT"NUMBER IS NEGATIVE"
ELSE
PRINT"NUMBER IS NEUTRAL"
END IF
END SUB
Wap to erase vowels from given string
CLS
INPUT"ENTER ANY STRING";A$
PRINT"STRING WITHOUT VOWELS =";ERA(A$)
END
FUNCTION ERA(A$)
FOR I = 1 TO LEN (A$)
B$=MID$(A$,I,1)
C$=UCASE$(B$)
IF C$<>"A" AND C$<>'E" AND C$<>"I" AND C$<>"O" AND C$<>"U" THEN D$=D$+C$
END IF
NEXT I
ERA=D$
END FUNCTION
Wap to check the character is capital or small
WAP TO DISPLAY 50, 42, 35, 29, 24 1O.......TERM
Wap to find the palindrome word
CLS
INPUT "ENTER ANY STRING"; S$
P$ = S$
IF P$ = REV$(S$) THEN
PRINT "THE GIVEN WORD IS PALINDROME "
ELSE
PRINT " THE GIVEN NO. IS NOT PALINDROME"
END IF
END
FUNCTION REV$ (S$)
FOR I = LEN(S$) TO 1 STEP -1
B$ = MID$(S$, I, 1)
W$ = W$ + B$
NEXT I
REV$ = W$
END FUNCTION
Wap To check whether the given no. is prime or composite
INPUT "ENTER ANY NUMBER"; N
CALL PRIME (N)
END
SUB PRIME (N)
C = 0
FOR I = 1 TO N
IF N MOD I = 0 THEN C = C + 1
NEXT I
IF C = 2 THEN
PRINT N; "IS PRIME NUMBER"
ELSE
PRINT N; "IS COMPOSITE NUMBER"
END IF
END SUB
Wap to find factorial of given no.
Wap to find whether the given no. is positive or negative
CLS
INPUT"ENTER ANY NO.";N
CALL CHECK(N$)
END
SUB CHECK(N)
IF N > 0 THEN
PRINT"POSITIVE NO."
ELSE IF N < 0 THEN
PRINT"NEGATIVE NO."
ELSE
PRINT"ZERO"
END IF
END SUB
Display 9,7,5....1
CLS
CALL SERIES
END
SUB SERIES()
FOR I = 9 TO 1 STEP-2
PRINT I
NEXT I
END SUB
Wap to calculate distance travelled by body
CLS
INPUT"ENTER VELOCITY";U
INPUT"ENTER TIME";T
INPUT"ENTER ACCELERATION";A
PRINT"DISTANCE TRAVELLED BY BODY IS ";DIS(U,T,A)
END
FUNCTION DIS(U,T,A)
DIS=U*T+1/2*A*T^2
END FUNCTION
Wap to print only vowels from given word
CLS
INPUT"ENTER ANY WORD";N$
CALL DIS(N$)
END
SUB DIS(N$)
FOR I = 1 TO LEN(N$)
B$=MID$(N$,I,1)
C$=UCASE$(B$)
IF C$="A" OR C$="E" OR C$="I" OR C$="O" OR C$="U" THEN
PRINT C$
NEXT I
END SUB
Wap to find volume of box
CLS
INPUT"ENTER LENGTH";L
INPUT"ENTER BREADTH";B
INPUT"ENTER HEIGHT";H
PRINT" VOLUME OF BOX";VOL(L,B,H)
END
FUNCTION VOL(L,B,H)
VOL=L*B*H
END FUNCTION
Wap to check whether the given no. is completely divisible by 13 or not
WAP TO FIND CIRCUMFERENCE OF CIRCLE
CLS
INPUT"ENTER RADIUS";R
CALL CIR(R)
END
SUB CIR(R)
C=2*22/7*R
PRINT"CIRCUMFERENCE OF CIRCLE IS ";C
END SUB
Wap to find area of box
CLS
INPUT"ENTER LENGTH";L
INPUT"ENTER BREADTH";B
INPUT"ENTER HEIGHT";H
PRINT"THE AREA OF BOX IS ";AR(L,B,H)
END
FUNCTION AREA(L,B,H)
AR=2*(L*H+B*H+L*B)
END FUNCTION
Wap to display greatest among 3 no
Wap to display 1,1,2,3,5,8.....upto 10th term
CLS
CALL SERIES
END
SUB SERIES()
A=1
B=1
FOR I = 1 TO 10
PRINT A;
PRINT B;
A=A+B
B=B+A
NEXT I
END SUB
Wap to print natural no. from 1 to 5
CLS
CALL SERIES()
END
SUB SERIES()
FOR I = 1 TO 5
PRINT I
NEXT I
END SUB
Wap to find simple intrest
CLS
INPUT"ENTER PRINCIPLE":P
INPUT"ENTER TIME";T
INPUT"ENTER RATE":R
PRINT"SIMPLE INTEREST= ";SI(P,T,R)
END
FUNCTION SI(P,T,R)
SI=(P*T*R)/100
END FUNCTION
Wap to convert temperature in celsius into fahrenheit
Wap to find sum of digits
CLS
INPUT"ENTER ANY NO.";N
CALL SUM(N)
END
SUB SU8M(N)
S=0
WHILE N<>0
R = N MOD 10
S = S+R
N = N\10
WEND
PRINT"SUM OF DIGITS IS ";S
END SUB
Wap to count total no. of consonant in given word
CLS
INPUT"ENTER ANY STRING";N$
PRINT"TOTAL NO. OF CONSONANTS";COUNT(N$)
END
FUNCTION COUNT(N$)
FOR I = 1 TO LEN(N$)
B$ = MID$(N$,I,1)
C$ = UCASE$(B$)
IF C$<>"A" OR C$<>"E" OR C$<>"I" OR C$<>"O" OR C$<>"U" THEN C= C+1
NEXT I
COUNT = C
END FUNCTION
Wap to print first ten numbers
CLS
CALL SERIES
END
SUB SERIES()
FOR I = 1 TO 10 STEP 2
PRINT I
NEXT I
END SUB
Wap to find volume of cylinder
CLS
INPUT"ENTER RADIUS";R
INPUT"ENTER HEIGHT";H
PRINT"VOLUME OF CYLINDER IS";VOL(R,H)
END
FUNCTION VOL(R,H)
VOL=22/7*R^2*H
END FUNCTION
Wap to find area of triangle
Wap to count total no. of vowels in a word
Wap to find area of 4 wall
CLS
INPUT"ENTER LENGTH";L
INPUT'"ENTER BREADTH";B
INPUT"ENTER HEIGHT";H
CALL AR(L,B,H)
END
SUB AR(L,B,H)
ARE=2*H*(L+B)
PRINT"AREA OF 4 WALLS =";ARE
END SUB
Wap to display reverse of input string
CLS
INPUT"ENTER STRING";N$
CALL REV$($)
END
SUB REV$(N$)
FOR I = LEN(N$) TO 1 STEP-1
B$ = MID$(N$,I,1)
C$= C$+B$
NEXT I
PRINT"REVERSE OF STRING";C$
END SUB
Monday, September 30, 2019
QBASIC PROGRAM TO COUNT TOTAL NO. OF WORDS IN SENTENCE
CLS
INPUT "ENTER ANY STRING"; S$
PRINT "TOTAL NO. OF WORDS= "; COUNT(S$)
END
FUNCTION COUNT (S$)
WC = 1
FOR I = 1 TO LEN(S$)
B$ = MID$(S$, I, 1)
IF B$ = " " THEN
WC = WC + 1
END IF
NEXT I
COUNT = WC
END FUNCTION
QBASIC PROGRAM TO FIND AREA OF CIRCLE USING SUB PROCEDURE.
QBASIC PROGRAM TO PRINT TOTAL NUMBER OF VOWELS IN A GIVEN WORD UISING SUB PROCEDURE.
OR C$ = “O” OR C$ = “U” THEN C = C + 1
QBASIC PROGRAM TO FIND AVERAGE OF THREE NUMBERS USING FUNCTION.
Saturday, August 31, 2019
Election commission visit
My father
of my family. A father is a person who has got the sole responsibility than others. So, my hero name is Mlangai Tamang. You might think what does this name means? This name meaning is Kaaley in Tamang language. Most people feel difficult to pronounce his name and pronounce it funnily. My father is a hardworking person. He was born in Sindhupalchowk district. In beginning his life was easy but when he was child my grandparents died, his life turned to hell. He was left lonely. He used to be bullied by his big father. So, he took the decision to leave the house. He came to Kathmandu. In Kathmandu he started spending his life in his friend home by taking care of a cow. He used to graze the cow. Later on, He was adopted by the same family. He was i
interested in music since childhood. He used to play the flute in childhood. So in this way he was spending his life. Then one a man took him and got him the job in Nepal telecom. As being a new and young worker he was hated by everyone. He was not even trained so he didn’t know what to do at first. Even nobody taught him. Then he started learning by just seeing how other workers do. He worked so hard. Every day he used to go to office and see other workers and learned. In this way he even learned working. Now he was fulfilled by knowledge.He had a family, a well-income job and a house to live.
So, then he was married to my mother. And after 1 year I was born. He used to teach me Basic English, Maths and Nepali in home before admitting me to school. Later on my sister came in this world. And our family was completed.
Love you Baba❤❤❤❤❤❤❤👨👧
Saturday, August 17, 2019
My Experience On Police Community Partnership
Sunday, July 21, 2019
Experience On The Monsoon Hike
Route |
Changu Narayan Temple - Trishuli Dada - Muhan Pokhari |
Date |
July 20, 2019 |
Duration |
5 hours |
Participants |
11 teachers (Rajan Acharya, Deepak Shrestha, Khem Paneru, Kamal Nepal, Mahendra Kurumbang, Bijay Pokhrel, Hira Shrestha, Aarati Thapa, Archana Shrestha, Sabina Shahi and Dristy Dhungana) & 49 Students (Sachi Tamang, Aryan Chakradhar, Ang Furwa Sherpa, Ankit Paneru, Priyanka karki, Sneha Adhikari, Aarati Dawadi, Anjali Rana Magar, Anil Gurung, Lakpa Sherpa, Subodh Dahal, Tshering Bhotiya, Nishan Chaudhary, Kopila Gamal, Sujan Bhattarai, Simson Limbu, Tshering D. Sherpa, Sushant Pandit, Sujan Magar, Samip Lingden, Rahul Sunuwar, Sunil Giri, Ritika Khadgi, Bigyan Karki, Basanta T. Magar, Aakriti Rai, Luisha Rai, Krishnaa Shrestha, Laxmi Basnet, Chanda Karki, Alisha Thapa, Prerana Panta, Ang Chotti Sherpa, Suraj Karki, Bipana Shrestha, Aarohi Dhungana, Swastika Thapa, Diggaj Baral, Gyandesh Tamang, Jenish Shrestha, Sonima Gole, Albert Tamang, Aalok Lawati, Ayup Rai, Sijan Dangol, Sonam Sherpa, Barun Thanet, Mingma D. Sherpa, Sushant Magar
|
Report by |
Sachi Tamang |
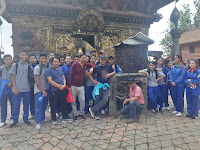
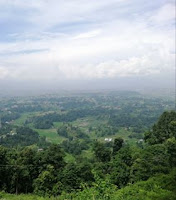
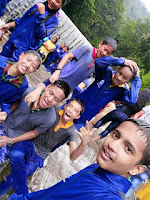
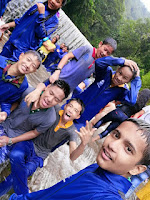
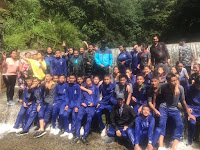
Sunday, June 16, 2019
Sericulture Development Center
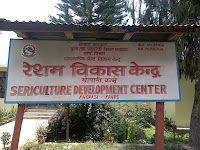
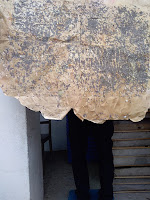
We, the students (Grade 10) of Jagat Mandir Secondary School were taken to the Sericulture Development Centre located at Khopasi, Kavre. We left the school at around 10 am for the destination. We travelled in the bus for around 1 hour. We clicked many photos in the bus. Most of us were busy using mobile phones. The weather was very hot that day. We were all sweating due to the hot temperature. I was clicking pictures in the bus. I also captured some comedy pictures of my friends. And finally, we reached to our destination. We reached there at around 11:30 am.
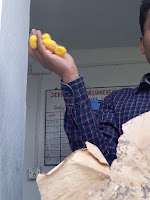
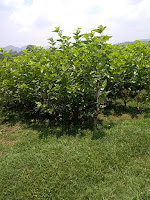
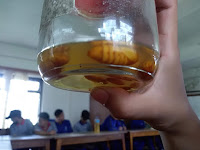
Saturday, May 18, 2019
My experience regarding the program related to Police-Community partnership
Sunday, May 12, 2019
Aandhiko Manoram Nritya
It was all about the problems that we are able to see in this stage known as teenage. It was really a nice drama I had ever seen. Being a teenager I got to know about various things related to us. The thing I loved in the drama was the presentation. We know being a teenager we have to face a lot of obstacles. We experience a kind of pressure from family, teachers and friends. We teenagers like to spend our time with peer groups rather than with our family. This is the age when teenagers start involving in bad things getting influenced by bad peer groups. Finally, the play ended. At last, the director of the play insisted our row to speak some words for the play. I was really nervous because I am not habitual to speak in front of such huge mass. One of the students of next school spoke about the knowledge she gained from drama. I and my friends in the same row insisted one of our friends to speak something about the drama. She has got a good speaking skill. So, she stood up and spoke very beautifully. That day I was really ashamed of myself but in the same way I was proud of my friend.
Thursday, January 17, 2019
Tour Experiences
Day 1
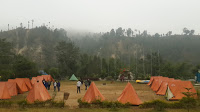
Then, we left for our destination. But the most surprising moment in the journey was we ate our meal on the same place where we had gone before year for the tour. We reached there around 9:38 am. We had our meal and left the camp at around 10:20 am.
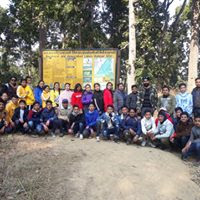
Day 2
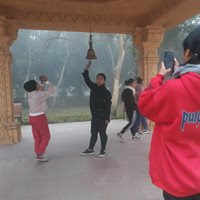
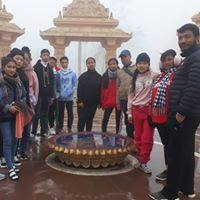
gumbas made by different countries. And the exciting moment was when we were informed that we were going to visit on Rickshaws. There were altogether 32 gumbas but we didn’t got chance to visit all of them. Then we went to visit the birthplace of Siddhartha Gautam. There we saw many tourists. Then after visiting, we again started our journey towards Butwal where we had to stay at night. We reached there at around 5-6 pm. Then we visited the sweets shop where we ate pedas. Then we started our journey towards our hotel in Butwal at 7:30. We reached there a bit late.
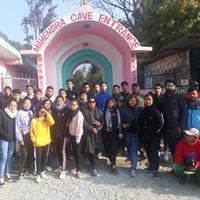
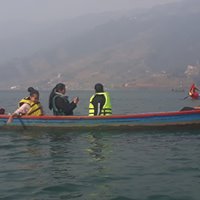
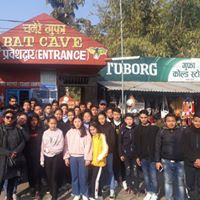
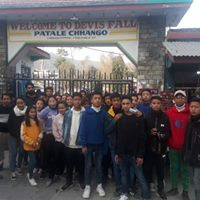
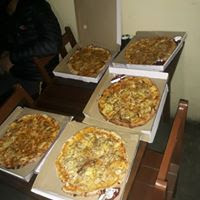
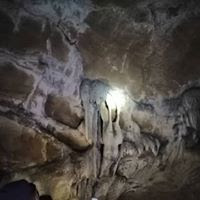
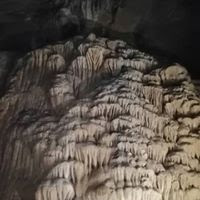
Experience of COVID-19 and the cancellation of SEE.
As we know, that this pandemic disease has started from the Wuhan district of China. This viruswvirus discovered out on 31st December 2019 ...
-
Each and every person have got a superhero, and for me its my Dad. My father Love U Dad name is Mlangai Tamang. He is a Technician. H...
-
My eyes fill with tear while recalling all those memories that I had made in JM. It has become 7 years since I have come to Jagat Mandir...